CSS Essential Training Notes
Referencing CSS
External CSS
HTML 5
<link rel="stylesheet" href="css/styles.css" />
HTML 4
<link rel="stylesheet" href="css/styles.css" type="text/css" />
@import
- Not recommended because of the potential of slowing down the website.
- Usually used in CSS Pre-processors.
@import url("b.css");
<head>
<style>
@import url("css/b.css");
</style>
</head>
Inline CSS
- Shouldn’t be used widely.
- Overrides defined stylesheets.
<p style="color:red;">Red text!</p>
Internal CSS
- Inefficient with multiple pages.
- Better than inline CSS.
<head>
<style>
h1 {
color: green;
}
</style>
</head>
وصلة للملاحظة الرئيسة
Referencing CSS
External CSS
HTML 5
<link rel="stylesheet" href="css/styles.css" />
HTML 4
<link rel="stylesheet" href="css/styles.css" type="text/css" />
@import
- Not recommended because of the potential of slowing down the website.
- Usually used in CSS Pre-processors.
@import url("b.css");
<head>
<style>
@import url("css/b.css");
</style>
</head>
Inline CSS
- Shouldn’t be used widely.
- Overrides defined stylesheets.
<p style="color:red;">Red text!</p>
Internal CSS
- Inefficient with multiple pages.
- Better than inline CSS.
<head>
<style>
h1 {
color: green;
}
</style>
</head>
Images and Pixel Density
- Images should be twice of the required size to be shown perfectly on high pixel density displays like Apple Retina.
- Background images should be in between of 1400px and 2000px width.
CSS Syntax
- Selector
- Declaration Block
- Declaration [property: value;]
CSS Values and Units
CSS Values and Units
Numeric
width: 80%;
height: 10em;
boarder-width: 5px;
animation-iteration-count: 3;
font-weight: 400;
font-size: 1.25rem;
transform: rotate(360deg);
animation-duration: 750ms;
Length datatype
Used to specify sizing with two types of units: absolute and relative.
Absolute
- Fixed unit.
- Not affected by values in relative elements.
- Examples:
- px: Pixel
- cm: Centimeter
- mm: Millimeter
- in: Inch
- pc: Pica (equal to 1/6 of an inch)
- pt: point (equal to 1/72 of an inch)
- width: 1000px;
Relative
- Relational sizing, not fixed.
- Dependent upon values declared in parent and ancestor elements.
- Example: em, rem, vw, vh.
- em: Represents inherited font-size of element.
- rem: Represents the font-size of the root element.
- 1vw = 1% of the width of the viewport.
- 1vh = 1% of the height of the viewport.
- vmin: Equal to the smaller of vw and vh.
- vmax: Equal to the larger of vw and vh.
Unitless Values
- Numbers only:
line-height: 1.25;
- Keywords:
color: red;
Function Values
transform: rotate(90deg);
width: calc(80% - 20px);
background-image: url("image.png");
وصلة للملاحظة الرئيسة
CSS Values and Units
CSS Values and Units
Numeric
width: 80%;
height: 10em;
boarder-width: 5px;
animation-iteration-count: 3;
font-weight: 400;
font-size: 1.25rem;
transform: rotate(360deg);
animation-duration: 750ms;
Length datatype
Used to specify sizing with two types of units: absolute and relative.
Absolute
- Fixed unit.
- Not affected by values in relative elements.
- Examples:
- px: Pixel
- cm: Centimeter
- mm: Millimeter
- in: Inch
- pc: Pica (equal to 1/6 of an inch)
- pt: point (equal to 1/72 of an inch)
- width: 1000px;
Relative
- Relational sizing, not fixed.
- Dependent upon values declared in parent and ancestor elements.
- Example: em, rem, vw, vh.
- em: Represents inherited font-size of element.
- rem: Represents the font-size of the root element.
- 1vw = 1% of the width of the viewport.
- 1vh = 1% of the height of the viewport.
- vmin: Equal to the smaller of vw and vh.
- vmax: Equal to the larger of vw and vh.
Unitless Values
- Numbers only:
line-height: 1.25;
- Keywords:
color: red;
Function Values
transform: rotate(90deg);
width: calc(80% - 20px);
background-image: url("image.png");
CSS Selectors
CSS Selectors
Type Selectors
- Matches element name.
h1 {...}
Universal Selectors
- Applies to all elements.
* {
border: 1px solid black;
}
Class Selectors
<p class="fancy intro">Fancy intro paragraph.</p>
<p class="fancy">Fancy paragraph.</p>
<p>Regular paragraph.</p>
.fancy {
font-style: italic;
}
.fancy {
...;
} /* only fancy styles */
.intro {
...;
} /* only intro styles *x/
/* only applies if "fancy" AND "intro" are present */
.fancy.intro {
...;
}
ID Selectors
- Add the ID attribute to the HTML element
- You define the value
- The value is the selector, starting with a # symbol
- Can only be used once per page.
<div 1d="container">
<p>Paragraph 1 in a header.</p>
</div>
/* Not valid */
<section id="contalner">
<p>Paragraph in a header.</p>
</section>
#container {
text-align: center;
}
Descendant Selectors
Creates matching patterns based on the relationship between nested elements
<!-- Selects only the paragraphs contained within a section */
section p { ... } -->
<section>
<p>...</p>
<!-- this -->
</section>
<p>...</p>
<!-- Selects only links, inside of a paragraph, inside of section
section p a { ... } -->
<section>
<p>There's a <a href="#">link</a> inside this paragraph.</p>
<!-- this -->
<p>Paragraph</p>
<a href="#">Link</a>
</section>
<a href="#">Link</a>
<section class="container">
<hl>Heading</hl>
<p>Paragraph with a <span>span</span>.</p>
</section>
.container hl {
}
.container span {
}
Grouping Selectors
/* applies to all hl elements */
hl {
...;
}
/* applies to any element with this class */
.class {
...;
}
/* applies to both hl and h2 elements */
hl,
h2 {
...;
}
/* applies to all of these elements */
hl,
h2,
.class,
#id {
...;
}
section hl,
h2 {
}
/* expands to */
section hl {
}
h2 {
}
<section hl,
section h2 {
}
/* expands to */
section hl {
}
section h2 {
}
Combining Selectors
<p class="fancy intro">Fancy intro paragraph</p>
/* only applies if "fancy" AND "intro" classes are included */
.fancy.intro {
...;
}
/* applies to any element with this class */
.intro {
color: blue;
}
/* only applies to a <p> element with this class */
p.intro {
font-size: 15px;
}
Specificity
Specificity determines how browsers decide which CSS rule takes precedence.
- Universal (*)
- Element (p)
- Class / pseudo-class (.example)
- ID (#example)
- Inline Styles
- !important
* {
color: lightblue;
}
p {
color: green;
}
.example {
color: black;
}
#example {
color: purple;
}
section p {
color: lightgreen; /* Won't override */
}
Specificity Calculator
Advanced Selectors
Child Combinator (>)
Only matches to direct child element
/* descendant selector */
parent child {
}
ancestor descendant {
}
/* child combinator */
parent > child {
}
Sibling Combinators (+, ~)
Adjacent Sibling Combinators (+)
<section>
<p>Sibling to heading.</p>
<hl>Heading</hl>
<p>Sibling to heading</p>
<!-- adjacent sibling -->
<p>Sibling to heading.</p>
</section>
hl + p {
}
General Sibling Combinator (~)
<section>
<p>Sibling to heading.</p>
<hl>Heading</hl>
<p>Sibling to heading</p>
<!-- general sibling -->
<p>Sibling to heading.</p>
<!-- general sibling -->
</section>
hl ~ p {
}
Pseudo-Class Selectors
<section>
<p>Paragraph.</p>
<!-- this one will display red-->
<p>Paragraph.</p>
</section>
<section class="example">
<p>Paragraph.</p>
<!-- this one will display red-->
<p>Paragraph.</p>
<p>Paragraph.</p>
<!-- this one will display green-->
</section>
a {
color: #ffe66d;
}
a:hover {
text-decoration: none;
}
p:first-child {
color: red;
}
.example p:last-child {
color: green;
}
p:first-child {
color: red;
}
p:first-of-type {
color: green;
}
<section>
<p>Paragraph.</p>
<!-- this one will display red-->
<p>Paragraph.</p>
</section>
<section class="example">
<hl>Heading</hl>
<p>Paragraph.</p>
<!-- this one will display green-->
</section>
وصلة للملاحظة الرئيسة
CSS Selectors
CSS Selectors
Type Selectors
- Matches element name.
h1 {...}
Universal Selectors
- Applies to all elements.
* {
border: 1px solid black;
}
Class Selectors
<p class="fancy intro">Fancy intro paragraph.</p>
<p class="fancy">Fancy paragraph.</p>
<p>Regular paragraph.</p>
.fancy {
font-style: italic;
}
.fancy {
...;
} /* only fancy styles */
.intro {
...;
} /* only intro styles *x/
/* only applies if "fancy" AND "intro" are present */
.fancy.intro {
...;
}
ID Selectors
- Add the ID attribute to the HTML element
- You define the value
- The value is the selector, starting with a # symbol
- Can only be used once per page.
<div 1d="container">
<p>Paragraph 1 in a header.</p>
</div>
/* Not valid */
<section id="contalner">
<p>Paragraph in a header.</p>
</section>
#container {
text-align: center;
}
Descendant Selectors
Creates matching patterns based on the relationship between nested elements
<!-- Selects only the paragraphs contained within a section */
section p { ... } -->
<section>
<p>...</p>
<!-- this -->
</section>
<p>...</p>
<!-- Selects only links, inside of a paragraph, inside of section
section p a { ... } -->
<section>
<p>There's a <a href="#">link</a> inside this paragraph.</p>
<!-- this -->
<p>Paragraph</p>
<a href="#">Link</a>
</section>
<a href="#">Link</a>
<section class="container">
<hl>Heading</hl>
<p>Paragraph with a <span>span</span>.</p>
</section>
.container hl {
}
.container span {
}
Grouping Selectors
/* applies to all hl elements */
hl {
...;
}
/* applies to any element with this class */
.class {
...;
}
/* applies to both hl and h2 elements */
hl,
h2 {
...;
}
/* applies to all of these elements */
hl,
h2,
.class,
#id {
...;
}
section hl,
h2 {
}
/* expands to */
section hl {
}
h2 {
}
<section hl,
section h2 {
}
/* expands to */
section hl {
}
section h2 {
}
Combining Selectors
<p class="fancy intro">Fancy intro paragraph</p>
/* only applies if "fancy" AND "intro" classes are included */
.fancy.intro {
...;
}
/* applies to any element with this class */
.intro {
color: blue;
}
/* only applies to a <p> element with this class */
p.intro {
font-size: 15px;
}
Specificity
Specificity determines how browsers decide which CSS rule takes precedence.
- Universal (*)
- Element (p)
- Class / pseudo-class (.example)
- ID (#example)
- Inline Styles
- !important
* {
color: lightblue;
}
p {
color: green;
}
.example {
color: black;
}
#example {
color: purple;
}
section p {
color: lightgreen; /* Won't override */
}
Specificity Calculator
Advanced Selectors
Child Combinator (>)
Only matches to direct child element
/* descendant selector */
parent child {
}
ancestor descendant {
}
/* child combinator */
parent > child {
}
Sibling Combinators (+, ~)
Adjacent Sibling Combinators (+)
<section>
<p>Sibling to heading.</p>
<hl>Heading</hl>
<p>Sibling to heading</p>
<!-- adjacent sibling -->
<p>Sibling to heading.</p>
</section>
hl + p {
}
General Sibling Combinator (~)
<section>
<p>Sibling to heading.</p>
<hl>Heading</hl>
<p>Sibling to heading</p>
<!-- general sibling -->
<p>Sibling to heading.</p>
<!-- general sibling -->
</section>
hl ~ p {
}
Pseudo-Class Selectors
<section>
<p>Paragraph.</p>
<!-- this one will display red-->
<p>Paragraph.</p>
</section>
<section class="example">
<p>Paragraph.</p>
<!-- this one will display red-->
<p>Paragraph.</p>
<p>Paragraph.</p>
<!-- this one will display green-->
</section>
a {
color: #ffe66d;
}
a:hover {
text-decoration: none;
}
p:first-child {
color: red;
}
.example p:last-child {
color: green;
}
p:first-child {
color: red;
}
p:first-of-type {
color: green;
}
<section>
<p>Paragraph.</p>
<!-- this one will display red-->
<p>Paragraph.</p>
</section>
<section class="example">
<hl>Heading</hl>
<p>Paragraph.</p>
<!-- this one will display green-->
</section>
CSS colors
- Keywords
Resource to get color keywords: http://colours.neilorangepeel.com.
color: red;
background-color: w;
- Hex values (case-sensitive):
#000000
- rgb:
rgb(0, 0, 0)
- rgba:
rgba(0, 0, 0, 1)
with alpha.
- hsl: define a color by hue, saturation and lightness.
hsl(270, 60%, 70%)
.
- Hue is specified as angle, while saturation, and lightness are percentages.
- https://css-tricks.com/examples/HSLaExplorer/
- hsla:
hsla(270, 60%, 70%, 0.7)
with alpha.
Generate color palettes
وصلة للملاحظة الرئيسة
CSS colors
- Keywords
Resource to get color keywords: http://colours.neilorangepeel.com.
color: red;
background-color: w;
- Hex values (case-sensitive):
#000000
- rgb:
rgb(0, 0, 0)
- rgba:
rgba(0, 0, 0, 1)
with alpha. - hsl: define a color by hue, saturation and lightness.
hsl(270, 60%, 70%)
.- Hue is specified as angle, while saturation, and lightness are percentages.
- https://css-tricks.com/examples/HSLaExplorer/
- hsla:
hsla(270, 60%, 70%, 0.7)
with alpha.
Generate color palettes
وصلة للملاحظة الرئيسةCascade
- Order matters, from top to bottom.
!important
Keyword overrides source order and specificity.
Pseudo-classes
- :link :visited :hover :active :focus
Inline block and display
- For inline elements, adding width and height has no effect.
- Adding
display: block
to an inline element will make it display like block elements. - Setting
display: inline
to a block element will give it the characteristics of inline elements. - There is one more value that you’ll probably use more often, which is
inline-block
. This will apply the characteristics of both.- The width and height values will be applied, just like block elements, but the items are displayed side by side, just like inline elements, and they only wrap when there’s no longer any space in the container.
- This is a good example of separating content from style.
- You may want your elements to display on a new line, or on the same line, but instead of picking an HTML element that looks a particular way, choose the most semantic element for the content, and then change the display with CSS.
CSS Box Model
The Box Model
- Content box
- Padding box
- Border box
- Margin box
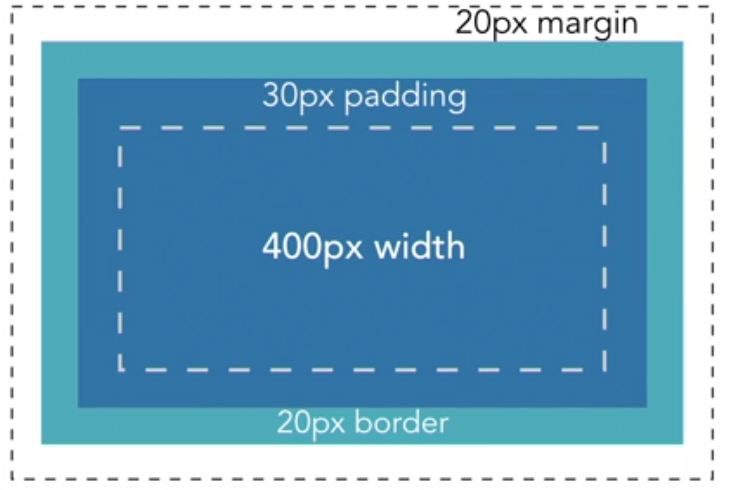
Example:
- 400 width
- 30 padding-left
- 30 padding-right
- 20 border-left
- 20 border-right
- 500px total width
Element height and width calculation
Final element width = left border + left padding + width + right padding + right border
Final element height = top border + top padding + height + bottom padding + bottom border
Box Properties
- Width
- Height
- Padding
- Margin
- Boarder
Width and Height
The width and height properties change the size of the content box. Inline elements require the display property.
/* Block element */
div {
width: 100px;
height: 100px;
}
/* inline-element */
span {
width: 100px;
height: 100px;
display: block;
/* OR */
display: inline-block;
}
Padding
Padding adds/removes space inside of the element but around the content box.
/* Longhand */
padding-top: 2px;
padding-right: 2px;
padding-bottom: 2px;
padding-left: 2px;
/* shorthand */
padding: 2px 2px 2px 2px; /* top right bottom left */
/* The shorthand syntax can use 1-4 values. */
padding: 2px; /* same on all sides */
padding: 2px 10px; /* top & bottom, right & left */
padding: 2px 10px 5px; /* top, right & left, bottom */
padding: 2px 10px 5px 2px; /* top, right, bottom, left */
/* You can also mix different types of length units. */
padding: 10px 2%; /* top & bottom, right & left */
/* valid */
padding: 0px;
padding: 0;
padding: 2px 0;
/* not valid */
padding: 2;
padding: -10;
Margin
Margin adds/removes space around the element.
/* longhand */
margin-top: 2px;
margin-right: 2px;
margin-bottom: 2px;
margin-left: 2px;
/* shorthand */
margin: 2px 2px 2px 2px; /* top right bottom left */
margin: 2px 10px 5px; /* top, right & left, bottom */
margin: 2px 10px; /* top & bottom, right & left +*/
margin: 2px; /* same on all sides */
Border
The border property displays a border between the margin and padding.
/* shorthand */
border: 2px solid red;
/* longhand */
border-width: 2px;
border-style: solid;
border-color: red;
/* width */
border-width: 10px;
border-width: thin;
border-width: medium;
border-width: thick;
/* color */
border-color: blue;
border-color: #000000;
/* style */
border-style: none;
border-style: hidden;
border-style: dotted;
border-style: dashed;
border-style: solid;
border-style: double;
border-style: groove;
border-style: ridge;
border-style: inset;
border-style: outset;
وصلة للملاحظة الرئيسة
CSS Box Model
The Box Model
- Content box
- Padding box
- Border box
- Margin box
Example:
- 400 width
- 30 padding-left
- 30 padding-right
- 20 border-left
- 20 border-right
- 500px total width
Element height and width calculation
Final element width = left border + left padding + width + right padding + right border
Final element height = top border + top padding + height + bottom padding + bottom border
Box Properties
- Width
- Height
- Padding
- Margin
- Boarder
Width and Height
The width and height properties change the size of the content box. Inline elements require the display property.
/* Block element */
div {
width: 100px;
height: 100px;
}
/* inline-element */
span {
width: 100px;
height: 100px;
display: block;
/* OR */
display: inline-block;
}
Padding
Padding adds/removes space inside of the element but around the content box.
/* Longhand */
padding-top: 2px;
padding-right: 2px;
padding-bottom: 2px;
padding-left: 2px;
/* shorthand */
padding: 2px 2px 2px 2px; /* top right bottom left */
/* The shorthand syntax can use 1-4 values. */
padding: 2px; /* same on all sides */
padding: 2px 10px; /* top & bottom, right & left */
padding: 2px 10px 5px; /* top, right & left, bottom */
padding: 2px 10px 5px 2px; /* top, right, bottom, left */
/* You can also mix different types of length units. */
padding: 10px 2%; /* top & bottom, right & left */
/* valid */
padding: 0px;
padding: 0;
padding: 2px 0;
/* not valid */
padding: 2;
padding: -10;
Margin
Margin adds/removes space around the element.
/* longhand */
margin-top: 2px;
margin-right: 2px;
margin-bottom: 2px;
margin-left: 2px;
/* shorthand */
margin: 2px 2px 2px 2px; /* top right bottom left */
margin: 2px 10px 5px; /* top, right & left, bottom */
margin: 2px 10px; /* top & bottom, right & left +*/
margin: 2px; /* same on all sides */
Border
The border property displays a border between the margin and padding.
/* shorthand */
border: 2px solid red;
/* longhand */
border-width: 2px;
border-style: solid;
border-color: red;
/* width */
border-width: 10px;
border-width: thin;
border-width: medium;
border-width: thick;
/* color */
border-color: blue;
border-color: #000000;
/* style */
border-style: none;
border-style: hidden;
border-style: dotted;
border-style: dashed;
border-style: solid;
border-style: double;
border-style: groove;
border-style: ridge;
border-style: inset;
border-style: outset;
Creating a Content Wrapper
Create a container for just the content.
<section class="work">
<div class="content-wrap">
<!/-- new content wrap div -->
<h2>Work Experience</h2>
</div>
<!-- close content-wrap div -->
</section>
.content-wrap {
width: 950px;
margin: 0 auto;
}
.work {
background: blue;
}
CSS Typography
Categories of Typefaces
- Script: have a hand-lettered look and tend to be harder to read in small sizes, all caps, or long blocks of text.
- Decorative: Since decorative fonts tend to have big personalities, they too are usually reserved for headings and decorative details when used for the web.
- Monospace: each character uses the same amount of horizontal space. Each character is the same width. Often used for displaying code.
- Serif: distinguished by small, decorative lines, usually at the top or at the bottom of the letters.
- Sans-serif: tend to be viewed as traditional and formal. Sans-serif typefaces do not have decorative lines, and are often thought of as contemporary and modern.
Changing the font-family
body {
font-family: Helvetica, Arial, sans-serif;
}
p {
font-family: Ahem!, sans-serif; /* not valid */
font-family: Ahem\!, sans-serif; /* escaped, valid */
font-family: "Ahem!", sans-serif; /* double quoted, valid */
font-family: "Ahem!", sans-serif; /* single quoted, valid */
font-family: "Gill Sans", sans-serif; /* valid and recommended */
font-family: Gill Sans, sans-serif; /* valid */
}
Generic font-family Names
- serif (serif fonts)
- sans-serif (sans-serif fonts)
- cursive (script or decorative fonts)
- fantasy (decorative fonts)
- monospace (monospace fonts)
Web-safe fonts: http://cssfontstack.com/
Font Weight
- 100 - Thin
- 200 - Extra Light (Ultra Light)
- 300 - Light
- 400 - Normal
- 500 - Medium
- 600 - Semi Bold (Demi Bold)
- 700 - Bold
- 800 - Extra Bold (Ultra Bold)
- 900 - Black (Heavy)
font-weight: 100; /* lightest or thinnest */
font-weight: 200;
font-weight: 300;
font-weight: 400;
font-weight: 500,
font-weight: 600;
font-weight: 700;
font-weight: 800;
font-weight: 900; /* darkest or thickest */
- Normal is equal to 400 and is also the default for body text
- Bold is equal to 700 and is the default for headings
Font Style
Used to add or remove an italic style. There are three values: italic, oblique,
and normal.
p {
font-style: italic; /* add italic style x*/
}
em {
font-style: normal; /* removes italic style */
}
Web Fonts
- Downloaded font files
- Included in your project files just like other files (i.e. images)
- Declare and link to font files using
@font-face
@font-face {
font-family: "My Font";
src: url(my-font.woff); /* relative path */
src: url(http://example.com/fonts/my-font.woff); /* absolute ath */
}
body {
font-family: "My Font", Helvetica, sans-serif;
}
URL of the font
/* valid */
src: url(my-font.woff);
src: url("my-font.woff");
src: url("my-font.woff");
src: url("my font.woff");
src: url(my\font.woff);
/* not valid */
src: url(my font.woff);
Cross-browser Compatibility
@font-face {
font-family: "MyWebFont";
src: url("webfont.eot"); /* IE9 Compat Modes */
src: url("webfont.eot?#1iefix") format("embedded-opentype"), /* IE6-IE8 */ url("webfont.woff2") format("woff2"),
/* Super Modern Browsers */ url("webfont.woff") format("woff"), /* Pretty Modern Browsers */ url("webfont.ttf")
format("truetype"), /* Safari, Android, 10S */ url("webfont.svg#svgFontName") format("svg"); /* Legacy i0S */
}
Font Size
font-size: px, em, rem
- Different units for specifying the font size
- Relative values are calculated based on the nearest ancestor element
- Absolute values are fixed and not affected by ancestor elements
font-size: px
- Screens are measured in pixels
- Absolute value, great for accuracy
- Use whole numbers, avoid decimals
- Browser default = 16px
font-size: em
- Named after the letter “m”
- Relative unit
- Can use whole numbers or decimal points
- 1em = inherited font-size
font-size: rem
- root em
- Relative unit
- Only relative to the root element (
<html>
)
- Not affected by parent or ancestor elements
Font shorthand
- font-style
- font-size
- font-weight
- font-family
- font-variant
- line-height
Note:
- Must include values for the font-size and font-family
- May optionally include values for font-style, font-variant, font-weight, and line-height
- Order matters! font-style, font-variant, and font-weight must precede font-size
- font-variant = normal or small-caps only
- font-size/line-height
- font-family must be the last value specified
/* shorthand */
font: italic small-caps bold 24px/1.5 Helvetica, sans-serif;
/* longhand */
font-style: 1italic;
font-variant: small-caps;
font-weight: bold;
font-size: 24px;
line-height: 1.5;
font-family: Helvetica, sans-serif;
text-decoration
/* shorthand x*/
text-decoration: underline red solid;
/* longhand x*/
text-decoration-line: underline;
text-decoration-color: red;
text-decoration-style: solid;
text-align
- The text-align property can be used to align content within a block element
- Add it to the HTML element itself or the parent element
text-align: start;
text-align: end;
text-align: left;
text-align: right;
text-align: center;
text-align: justify;
text-align: justify-all;
text-align: match-parent;
line-height
- The line-height property sets the height of the space between two lines of text
- Closely related to font-size
- Can use different value types (px, %, ems, rems, or unitless)
text-transform
- The text-transform CSS property specifies how to capitalize an element’s text.
- It can be used to make text appear in all-uppercase or all-lowercase, or with each word capitalized.
text-transform: none;
text-transform: capitalize;
text-transform: uppercase;
text-transform: lowercase;
text-transform: full-width;
text-transform: full-size-kana;
وصلة للملاحظة الرئيسة
CSS Typography
Categories of Typefaces
- Script: have a hand-lettered look and tend to be harder to read in small sizes, all caps, or long blocks of text.
- Decorative: Since decorative fonts tend to have big personalities, they too are usually reserved for headings and decorative details when used for the web.
- Monospace: each character uses the same amount of horizontal space. Each character is the same width. Often used for displaying code.
- Serif: distinguished by small, decorative lines, usually at the top or at the bottom of the letters.
- Sans-serif: tend to be viewed as traditional and formal. Sans-serif typefaces do not have decorative lines, and are often thought of as contemporary and modern.
Changing the font-family
body {
font-family: Helvetica, Arial, sans-serif;
}
p {
font-family: Ahem!, sans-serif; /* not valid */
font-family: Ahem\!, sans-serif; /* escaped, valid */
font-family: "Ahem!", sans-serif; /* double quoted, valid */
font-family: "Ahem!", sans-serif; /* single quoted, valid */
font-family: "Gill Sans", sans-serif; /* valid and recommended */
font-family: Gill Sans, sans-serif; /* valid */
}
Generic font-family Names
- serif (serif fonts)
- sans-serif (sans-serif fonts)
- cursive (script or decorative fonts)
- fantasy (decorative fonts)
- monospace (monospace fonts)
Web-safe fonts: http://cssfontstack.com/
Font Weight
- 100 - Thin
- 200 - Extra Light (Ultra Light)
- 300 - Light
- 400 - Normal
- 500 - Medium
- 600 - Semi Bold (Demi Bold)
- 700 - Bold
- 800 - Extra Bold (Ultra Bold)
- 900 - Black (Heavy)
font-weight: 100; /* lightest or thinnest */
font-weight: 200;
font-weight: 300;
font-weight: 400;
font-weight: 500,
font-weight: 600;
font-weight: 700;
font-weight: 800;
font-weight: 900; /* darkest or thickest */
- Normal is equal to 400 and is also the default for body text
- Bold is equal to 700 and is the default for headings
Font Style
Used to add or remove an italic style. There are three values: italic, oblique, and normal.
p {
font-style: italic; /* add italic style x*/
}
em {
font-style: normal; /* removes italic style */
}
Web Fonts
- Downloaded font files
- Included in your project files just like other files (i.e. images)
- Declare and link to font files using
@font-face
@font-face {
font-family: "My Font";
src: url(my-font.woff); /* relative path */
src: url(http://example.com/fonts/my-font.woff); /* absolute ath */
}
body {
font-family: "My Font", Helvetica, sans-serif;
}
URL of the font
/* valid */
src: url(my-font.woff);
src: url("my-font.woff");
src: url("my-font.woff");
src: url("my font.woff");
src: url(my\font.woff);
/* not valid */
src: url(my font.woff);
Cross-browser Compatibility
@font-face {
font-family: "MyWebFont";
src: url("webfont.eot"); /* IE9 Compat Modes */
src: url("webfont.eot?#1iefix") format("embedded-opentype"), /* IE6-IE8 */ url("webfont.woff2") format("woff2"),
/* Super Modern Browsers */ url("webfont.woff") format("woff"), /* Pretty Modern Browsers */ url("webfont.ttf")
format("truetype"), /* Safari, Android, 10S */ url("webfont.svg#svgFontName") format("svg"); /* Legacy i0S */
}
Font Size
font-size: px, em, rem
- Different units for specifying the font size
- Relative values are calculated based on the nearest ancestor element
- Absolute values are fixed and not affected by ancestor elements
font-size: px
- Screens are measured in pixels
- Absolute value, great for accuracy
- Use whole numbers, avoid decimals
- Browser default = 16px
font-size: em
- Named after the letter “m”
- Relative unit
- Can use whole numbers or decimal points
- 1em = inherited font-size
font-size: rem
- root em
- Relative unit
- Only relative to the root element (
<html>
) - Not affected by parent or ancestor elements
Font shorthand
- font-style
- font-size
- font-weight
- font-family
- font-variant
- line-height
Note:
- Must include values for the font-size and font-family
- May optionally include values for font-style, font-variant, font-weight, and line-height
- Order matters! font-style, font-variant, and font-weight must precede font-size
- font-variant = normal or small-caps only
- font-size/line-height
- font-family must be the last value specified
/* shorthand */
font: italic small-caps bold 24px/1.5 Helvetica, sans-serif;
/* longhand */
font-style: 1italic;
font-variant: small-caps;
font-weight: bold;
font-size: 24px;
line-height: 1.5;
font-family: Helvetica, sans-serif;
text-decoration
/* shorthand x*/
text-decoration: underline red solid;
/* longhand x*/
text-decoration-line: underline;
text-decoration-color: red;
text-decoration-style: solid;
text-align
- The text-align property can be used to align content within a block element
- Add it to the HTML element itself or the parent element
text-align: start;
text-align: end;
text-align: left;
text-align: right;
text-align: center;
text-align: justify;
text-align: justify-all;
text-align: match-parent;
line-height
- The line-height property sets the height of the space between two lines of text
- Closely related to font-size
- Can use different value types (px, %, ems, rems, or unitless)
text-transform
- The text-transform CSS property specifies how to capitalize an element’s text.
- It can be used to make text appear in all-uppercase or all-lowercase, or with each word capitalized.
text-transform: none;
text-transform: capitalize;
text-transform: uppercase;
text-transform: lowercase;
text-transform: full-width;
text-transform: full-size-kana;
CSS Layouts Float and Position
CSS Layouts Float and Position
Float
The float property can be used to change the normal document flow by floating elements to the left or right side of its container. And when elements are floated, they are removed from the normal flow
though they remain part of the flow, resulting in the change of the positioning of the surrounding elements.
img {
float: left;
margin: 10px;
}
-
This will place the image to the left side of its container.
-
The text in the following paragraphs will fill the available area and line up right to the edge of the image.
-
We can add some margin in to create a little space between the image and the text.
-
By putting the background color on the paragraphs, it’s now easier to see that while the text is flowing around the image, the boundaries of the element is actually behind the image.
p {
background: #cdcdcd;
padding: 10px;
}
- Floated elements are taken out of the normal flow, so the other elements move up to fill the space as if the floated element was no longer there.
- But the content of those elements will still make room for the floated element and fill up the remaining space around it.
Clearing the Float
- You can clear the float using the
clear
property.
- The values for clear can be
left
or right
to specifically clear a left or right float, but I like to use the third option, both
, which clears left and right floats.
.clear {
clear: both; /* clears left and right floats */
}
What if there’s no element to apply the clear to?
Option 1: overflow
Overflow is actually used to specify how to display content that doesn’t fit in its container.
Different overflow values can be used to determine how to display the overflow of content.
hidden
clips the overflow of content, this may be used for decorative content, like an image, but isn’t very useful for text since clipped content can’t be accessed.
auto
adds a scroll bar, but only when there is overflowing content.
scroll
will always show a scroll bar on the X and Y axis, even when the content does not flow outside of its container.
<div class="parent">
<div class="floated">...</div>
<div class="floated">...</div>
</div>
.floated {
float: left;
}
.parent {
overflow: hidden;
/* OR */
overflow: auto;
}
Option 2: “clearfix” Hack
CSS snippet added to the parent of floated elements.
- This group of CSS styles need to be added to the parent of the floated elements.
- When adding the class to the HTML, do not include the colon after.
- This is a pseudo-element and is used in conjunction with another selector.
- This fix has been created and maintained by the developer community, and if you’re curious, the history of this fix can be found in this CSS tricks article.
<div class="clearfix">
<p>floated element</p=>
<p>floated element</p>
</div>
.clearfix:after {
content: "";
display: table;
clear: both;
}
Option 3: display
Not currently supported in all browsers.
- A newer way is in the works to solve the problem, using the display property with a value of flow-root.
- While this provides a solution, it is not currently supported by all modern browsers, and is still in the draft phase.
<div class="parent">
<div>floated element</div>
<div>floated element</div>
</div>
.parent {
display: flow-root;
}
box-sizing
box-sizing: content-box
- The initial box-sizing value is content-box.
- Width and height values change the size of the content-box.
- But when padding and border styles are added, they increase the size of the elements, adding to the width and height of the content box.
- Margin just adds the space around the element.
box-sizing: border-box
- Using the border-box value instead, we’ll include the padding and border space within the dimensions set with the width and height properties.
- This will make the content-box smaller, but the overall element will maintain the same width and height values without any adjustments.
The Box Model Fix
- This CSS Layouts Float and Position > box-sizing border-box is often referred to as the box model fix.
- To use this fix, add this CSS code snippet to all of your projects.
- More Resources: 1, 2.
/* border box fix */
html {
box-sizing: border-box;
}
*,
*:before,
*:after {
box-sizing: inherit;
}
Position
The position property can also be used to change the flow of the document. There are 5 different values. Each responsible for a different type of positioning.
static
: not positioned
relative
: relative to current position
absolute
: relative to containing element
fixed
: relative to the viewport
sticky
: relative to containing element and viewport
Position is also used with a combination of the top, right, bottom, and left properties.
.box {
position: relative;
top: 10px;
right: 10px;
}
Z-Index
- Every time an HTML element is added within the same container, the elements are stacked in layers on the Z axis.
- An element with a higher stack level is rendered in front of the element with the lower stack level.
- Most of the time, it’s hard to see how the elements stack on the Z index, unless they overlap each other.
Default stacking order
- HTML > floated > in-line > positioned.
- We can use the Z index property to change this, but it only works if the element has some type of position applied to it.
<div class="block1">block 1</div>
<div class="block2">block 2</div>
<div class="float">
<p>float</p>
<span class="inline">inline</span>
</div>
<div class="position">position</div>
/* root element */
html {
background: gray;
}
/* viewport */
body {
background: white;
height: 400px;
}
.block1 {
background: pink;
position: relative;
z-index: 100;
}
.block2 {
background: red;
margin: -60px 0 0 20px;
}
.float {
background: lightblue;
float: left;
margin: -150px 0 0 100px;
}
.inline {
background: blue;
color: white;
padding: 10px 40px 10px 10px;
}
.position {
background: orange;
position: relative;
left: 180px;
top: -165px;
height: 110px;
}
/* shared div styles */
div {
padding: 20px;
width: 200px;
height: 80px;
}
وصلة للملاحظة الرئيسة
CSS Layouts Float and Position
CSS Layouts Float and Position
Float
The float property can be used to change the normal document flow by floating elements to the left or right side of its container. And when elements are floated, they are removed from the normal flow though they remain part of the flow, resulting in the change of the positioning of the surrounding elements.
img {
float: left;
margin: 10px;
}
-
This will place the image to the left side of its container.
-
The text in the following paragraphs will fill the available area and line up right to the edge of the image.
-
We can add some margin in to create a little space between the image and the text.
-
By putting the background color on the paragraphs, it’s now easier to see that while the text is flowing around the image, the boundaries of the element is actually behind the image.
p {
background: #cdcdcd;
padding: 10px;
}
- Floated elements are taken out of the normal flow, so the other elements move up to fill the space as if the floated element was no longer there.
- But the content of those elements will still make room for the floated element and fill up the remaining space around it.
Clearing the Float
- You can clear the float using the
clear
property. - The values for clear can be
left
orright
to specifically clear a left or right float, but I like to use the third option,both
, which clears left and right floats.
.clear {
clear: both; /* clears left and right floats */
}
What if there’s no element to apply the clear to?
Option 1: overflow
Overflow is actually used to specify how to display content that doesn’t fit in its container.
Different overflow values can be used to determine how to display the overflow of content.
hidden
clips the overflow of content, this may be used for decorative content, like an image, but isn’t very useful for text since clipped content can’t be accessed.auto
adds a scroll bar, but only when there is overflowing content.scroll
will always show a scroll bar on the X and Y axis, even when the content does not flow outside of its container.
<div class="parent">
<div class="floated">...</div>
<div class="floated">...</div>
</div>
.floated {
float: left;
}
.parent {
overflow: hidden;
/* OR */
overflow: auto;
}
Option 2: “clearfix” Hack
CSS snippet added to the parent of floated elements.
- This group of CSS styles need to be added to the parent of the floated elements.
- When adding the class to the HTML, do not include the colon after.
- This is a pseudo-element and is used in conjunction with another selector.
- This fix has been created and maintained by the developer community, and if you’re curious, the history of this fix can be found in this CSS tricks article.
<div class="clearfix">
<p>floated element</p=>
<p>floated element</p>
</div>
.clearfix:after {
content: "";
display: table;
clear: both;
}
Option 3: display
Not currently supported in all browsers.
- A newer way is in the works to solve the problem, using the display property with a value of flow-root.
- While this provides a solution, it is not currently supported by all modern browsers, and is still in the draft phase.
<div class="parent">
<div>floated element</div>
<div>floated element</div>
</div>
.parent {
display: flow-root;
}
box-sizing
box-sizing: content-box
- The initial box-sizing value is content-box.
- Width and height values change the size of the content-box.
- But when padding and border styles are added, they increase the size of the elements, adding to the width and height of the content box.
- Margin just adds the space around the element.
box-sizing: border-box
- Using the border-box value instead, we’ll include the padding and border space within the dimensions set with the width and height properties.
- This will make the content-box smaller, but the overall element will maintain the same width and height values without any adjustments.
The Box Model Fix
- This CSS Layouts Float and Position > box-sizing border-box is often referred to as the box model fix.
- To use this fix, add this CSS code snippet to all of your projects.
- More Resources: 1, 2.
/* border box fix */
html {
box-sizing: border-box;
}
*,
*:before,
*:after {
box-sizing: inherit;
}
Position
The position property can also be used to change the flow of the document. There are 5 different values. Each responsible for a different type of positioning.
static
: not positionedrelative
: relative to current positionabsolute
: relative to containing elementfixed
: relative to the viewportsticky
: relative to containing element and viewport
Position is also used with a combination of the top, right, bottom, and left properties.
.box {
position: relative;
top: 10px;
right: 10px;
}
Z-Index
- Every time an HTML element is added within the same container, the elements are stacked in layers on the Z axis.
- An element with a higher stack level is rendered in front of the element with the lower stack level.
- Most of the time, it’s hard to see how the elements stack on the Z index, unless they overlap each other.
Default stacking order
- HTML > floated > in-line > positioned.
- We can use the Z index property to change this, but it only works if the element has some type of position applied to it.
<div class="block1">block 1</div>
<div class="block2">block 2</div>
<div class="float">
<p>float</p>
<span class="inline">inline</span>
</div>
<div class="position">position</div>
/* root element */
html {
background: gray;
}
/* viewport */
body {
background: white;
height: 400px;
}
.block1 {
background: pink;
position: relative;
z-index: 100;
}
.block2 {
background: red;
margin: -60px 0 0 20px;
}
.float {
background: lightblue;
float: left;
margin: -150px 0 0 100px;
}
.inline {
background: blue;
color: white;
padding: 10px 40px 10px 10px;
}
.position {
background: orange;
position: relative;
left: 180px;
top: -165px;
height: 110px;
}
/* shared div styles */
div {
padding: 20px;
width: 200px;
height: 80px;
}
Checking Browser Support
- Can I use is a great reference for checking browser support.
- Just do a search for any CSS property, and the search results will show you the browser support.
- There is also additional information, such as known issues or any additional resources.
CSS Layouts Flexbox and Grid
CSS Layouts Flexbox and Grid
- Both Flexbox and Grid are new layout modules that can be used to create layouts that are more advanced than previous techniques.
- While they share some similar syntax and some of the same properties, they each have their own specialties.
- With Flexbox, items are aligned on a single axis. It is often described as being one-dimensional. While you can set the main axis to arrange items as rows or columns, Flexbox only deals with one dimension at time.
- Grid layouts are thought of as two-dimensional, because it can arrange items into rows and columns at the same time.
- While both of these methods can be used for layouts, Flexbox is great for space distribution of items within the same axis. Grid is ideal for layouts that require more control with rows and columns.
Flexbox
https://developer.mozilla.org/en-US/docs/Web/CSS/flex
- flex container: the parent element
- flex items: the child elements
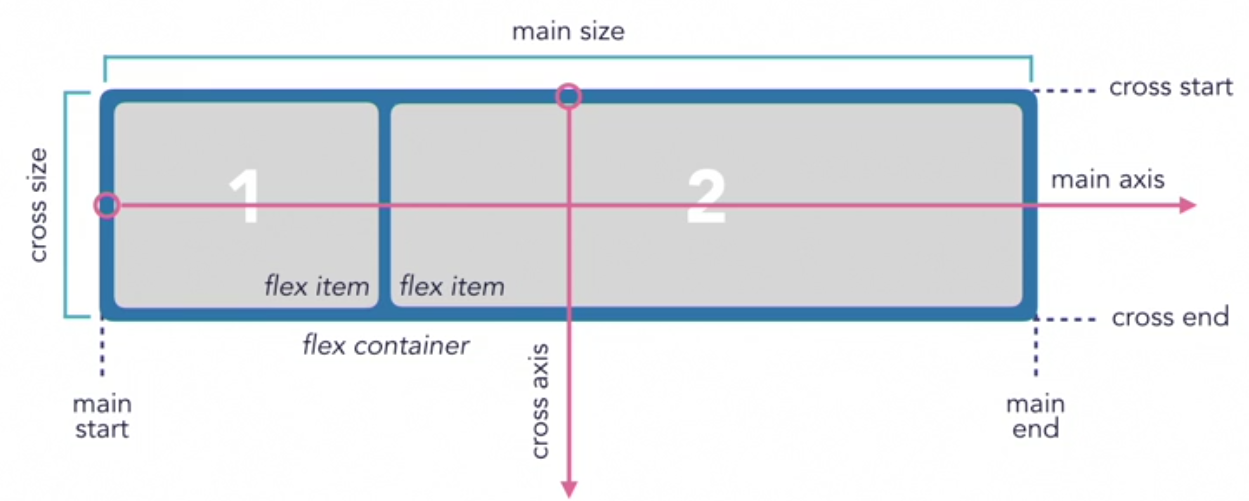
flex-direction
- Determines the direction of the main axis
There are four values: row
, row-reverse
, column
and column-reverse
.
flex-flow Shorthand
/* shorthand */
flex-flow: column wrap;
/* longhand */
flex-direction: column;
flex-wrap: wrap;
<section class="flex-container">
<div>
flex item
<div>not a flex item</div>
</div>
<div>flex item</div>
<div>flex 1tem</div>
<div>flex item</div>
</section>
.flex-container {
background: lightgray;
margin-bottom: 10px;
width: 500px;
height: 200px;
display: flex;
/* OR */
display: inline-flex;
flex-wrap: wrap;
/* OR */
flex-wrap: nowrap;
/* OR */
flex-wrap: wrap-reverse;
flex-direction: column;
}
.flex-item {
box-sizing: border-box;
border: 1px solid blue;
padding: 10px;
width: 100px;
}
flex-
Properties
These must be applied to the flex items directly not to the container.
flex-basis
: sets the initial size of the flex-items
flex-grow
: determines how items will expand if there is extra space in the container
flex-shrink
: determines how items will shrink if there isn’t enough space in the container
flex Shorthand
flex: grow shrink basis;
flex: 0 1 100px;
If you wanted the items to be sized differently, then flex must be applied to the individual flex items.
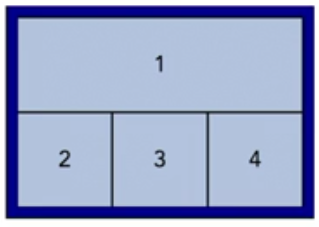
<div class="flex-container">
<div class="flex-item-first">1</div>
<div class="flex-item">2</div>
<div class="flex-item">3</div>
<div class="flex-item">4</div>
</div>
.flex-container {
display: flex;
}
.flex-item-first {
flex: 0 0 100%;
}
.flex-item {
flex: 1 1 auto;
}
Alignment
- justify-content aligns items on the main axis
- align-items aligns items on the cross axis
.box {
display: flex;
align-items: center;
justify-content: center;
}
/* Basic keywords */
align-items: normal;
align-items: stretch;
/* Positional alignment */
/* align-items does not take left and right values */
align-items: center; /* Pack items around the center */
align-items: start; /* Pack items from the start */
align-items: end; /* Pack items from the end */
align-items: flex-start; /* Pack flex items from the start */
align-items: flex-end; /* Pack flex items from the end */
/* Positional alignment */
justify-content: center; /* Pack items around the center */
justify-content: start; /* Pack items from the start */
justify-content: end; /* Pack items from the end */
justify-content: flex-start; /* Pack flex items from the start */
justify-content: flex-end; /* Pack flex items from the end */
justify-content: left; /* Pack items from the left */
justify-content: right; /* Pack items from the right */
/* Baseline alignment */
/* justify-content does not take baseline values */
/* Normal alignment */
justify-content: normal;
/* Distributed alignment */
justify-content: space-between; /* Distribute items evenly
The first item is flush with the start,
the last is flush with the end */
justify-content: space-around; /* Distribute items evenly
Items have a half-size space
on either end */
justify-content: space-evenly; /* Distribute items evenly
Items have equal space around them */
justify-content: stretch; /* Distribute items evenly
Stretch 'auto'-sized items to fit
the container */
Grid
https://www.mozilla.org/en-US/developer/css-grid/
-
Grids are broken down into evenly spaced columns and rows that are used as a guide for laying out page components.
-
Gutters are often included to add consistent spacing between columns.
-
Page components are arranged within the columns.
-
grid container - the parent element.
-
grid items — the child elements within the grid container
<div class="grid-container">
<div class="grid-item">
<div>Not a grid item</div>
</div>
<div class="grid-item"></div>
<div class="grid-item"></div>
</div>
.grid-container {
display: grid;
/* OR */
display: inline-grid;
}
-
When using the value of grid, unless specified, the items will span the width of its container.
-
When using the value of grid, the container will be displayed as block-level element and stacked on top of other block element.
-
When using the value of inline-grid, the items will span the width of its content.
-
When using the value of inline-grid, the whole container will display inline next to other inline containers.
-
Grid lines are the horizontal and vertical lines that divide the grid into columns and rows.
- Grid lines are also used to determine the position of the grid items and are referred to by a numerical index, or by custom name.
- When using a numerical index, both the vertical and horizontal grid lines start at one.
- Grid lines can also be referred to by a negative numerical index which allows you to reference the opposite end of the grid.
-
A grid cell refers to a single unit, defined by where the grid row and column intersect.
- The space between two adjacent grid lines is the grid track, which is basically the columns and rows.
-
Grid tracks can optionally be separated by a gutter.
Explicit Grid
- To define a specific number of grid lines and tracks, use
grid-template-columns
and grid-template-rows
. This will create an explicit grid.
.grid-container {
display: grid;
grid-template-columns: 100px 100px 100px; /* 3 columns */
grid-template-rows: 100px 100px; /* 2 rows */
}
The Fraction Unit: Fr
grid-template-columns: 1fr 1fr 1fr;
grid-template-columns: 1fr 2fr 1fr;
grid-template-columns: mixmax(200px, 1fr) 2fr 1fr; /* Set minimum allowed size */
repeat()
- We can also define multiple tracks using another value, the repeat function.
- Instead of writing 1 fr three times, include the number of tracks, three in this example, followed by a comma and the size of the track such as 1 fr within the parentheses.
repeat([number of tracks], [size of tracks])
grid-template-columns: 1fr 1fr 1fr;
/* same as */
grid-template-columns: repeat(3, 1fr);
grid-template-columns: 50px repeat(2, 1fr);
Adding Gutters with gap
gap: 10px; /* rows & columns */
gap: 10px 20px; /* rows | columns */
/* valid */
gap: 20px;
gap: 16%;
gap: calc(19% + 20px)
/* not valid */
gap: 1fr
/* shorthand */
gap: 110px 20px;
/* longhand */
row-gap: 10px;
column-gap: 20px;
- The original grid layout specification included the grid-gap, grid-row-gap and grid-column-gap properties.
- It has been updated to gap to be used for both grid and Flexbox.
/* shorthand */
gap = grid-gap
/* longhand */
row-gap = grid-row-gap
column-gap = grid-column-gap
Implicit Grid
- When using a grid layout, grid template rows and grid template columns are used to define a fixed number of tracks to form an explicit grid. But sometimes you may have more grid items than the number of explicit tracks.
- Once an explicit grid is filled or if no explicit grid has been defined, the grid container will generate implicit grid tracks.
- If you don’t know how many items your grid needs to display you can create the whole grid using only an implicit grid, and if you know the minimum amount of grid items you could use an explicit and implicit grid together.
grid-auto-columns grid-auto-rows .grid-container {
display: grid;
grid-auto-columns: repeat(3, 1fr); /* 3 columns */
grid-auto-rows: 100px 100px; /* 2 rows */
}
Defining the grid Structure
.grid-container {
display: grid;
grid-template-columns: 100px repeat(2, 1fr);
grid-template-rows: 100px;
grid-auto-rows: 200px;
grid-auto-columns: 50px;
}
Positioning grid Items
grid-column = grid-column-start grid-column-end grid-row = grid-row-start grid-row-end .grid-item-1 {
grid-column-start: 2;
grid-column-end: 4;
/* shorthand */
grid-column: 2 / 4;
grid-column: 1 / -1; /* span from start */
grid-row-start: 1;
grid-row-end: 3;
/*shorthand */
grid-row: 1 / 3;
grid-row: 1 / span 3;
}
Grid Lines and grid-column
.grid-item-1 {
grid-column-start: 2;
grid-column-end: 4;
/* shorthand */
grid-column: 2 / 4;
}
.grid-item-1 {
grid-column-start: 2;
grid-column-end: 4;
grid-row-start: 1;
grid-row-end: 3;
/* shorthand */
grid-column: 2 / 4;
grid-row: 1 / 3;
}
Alignment
- justify-items defines the default justify-self for all items of the box, giving them all a default way of justifying each box along the appropriate axis.
- align-items aligns items on the Block Axis within their grid area.
- place-items is shorthand property allows you to align items along both the block and inline directions at once (align-items and justify-items properties).
.grid {
display: grid;
grid-template-columns: repeat(auto-fill, 50px);
align-items: center;
justify-content: center;
justify-items: stretch;
}
- justify-content aligns items on the inline axis of a grid container.
- align-content sets the distribution of space between and around content items along grid’s block axis.
- place-content is shorthand property allows you to align content along both the block and inline directions at once (align-items and justify-items properties).
Naming the grid columns/rows
body {
grid-template-columns: [left] 1fr [middle] 1fr [right] 1fr;
}
header {
grid-column: left / right;
}
grid-template-areas
The grid-template-areas CSS property specifies named grid areas, establishing the cells in the grid and assigning them names.
<section id="page">
<header>Header</header>
<nav>Navigation</nav>
<main>Main area</main>
<footer>Footer</footer>
</section>
#page {
display: grid;
width: 100%;
height: 250px;
grid-template-areas:
"head head"
"nav main"
"nav foot";
grid-template-rows: 50px 1fr 30px;
grid-template-columns: 150px 1fr;
}
#page > header {
grid-area: head;
background-color: #8ca0ff;
}
#page > nav {
grid-area: nav;
background-color: #ffa08c;
}
#page > main {
grid-area: main;
background-color: #ffff64;
}
#page > footer {
grid-area: foot;
background-color: #8cffa0;
}
وصلة للملاحظة الرئيسة
CSS Layouts Flexbox and Grid
CSS Layouts Flexbox and Grid
- Both Flexbox and Grid are new layout modules that can be used to create layouts that are more advanced than previous techniques.
- While they share some similar syntax and some of the same properties, they each have their own specialties.
- With Flexbox, items are aligned on a single axis. It is often described as being one-dimensional. While you can set the main axis to arrange items as rows or columns, Flexbox only deals with one dimension at time.
- Grid layouts are thought of as two-dimensional, because it can arrange items into rows and columns at the same time.
- While both of these methods can be used for layouts, Flexbox is great for space distribution of items within the same axis. Grid is ideal for layouts that require more control with rows and columns.
Flexbox
https://developer.mozilla.org/en-US/docs/Web/CSS/flex
- flex container: the parent element
- flex items: the child elements
flex-direction
- Determines the direction of the main axis
There are four values: row
, row-reverse
, column
and column-reverse
.
flex-flow Shorthand
/* shorthand */
flex-flow: column wrap;
/* longhand */
flex-direction: column;
flex-wrap: wrap;
<section class="flex-container">
<div>
flex item
<div>not a flex item</div>
</div>
<div>flex item</div>
<div>flex 1tem</div>
<div>flex item</div>
</section>
.flex-container {
background: lightgray;
margin-bottom: 10px;
width: 500px;
height: 200px;
display: flex;
/* OR */
display: inline-flex;
flex-wrap: wrap;
/* OR */
flex-wrap: nowrap;
/* OR */
flex-wrap: wrap-reverse;
flex-direction: column;
}
.flex-item {
box-sizing: border-box;
border: 1px solid blue;
padding: 10px;
width: 100px;
}
flex-
Properties
These must be applied to the flex items directly not to the container.
flex-basis
: sets the initial size of the flex-itemsflex-grow
: determines how items will expand if there is extra space in the containerflex-shrink
: determines how items will shrink if there isn’t enough space in the container
flex Shorthand
flex: grow shrink basis;
flex: 0 1 100px;
If you wanted the items to be sized differently, then flex must be applied to the individual flex items.
<div class="flex-container">
<div class="flex-item-first">1</div>
<div class="flex-item">2</div>
<div class="flex-item">3</div>
<div class="flex-item">4</div>
</div>
.flex-container {
display: flex;
}
.flex-item-first {
flex: 0 0 100%;
}
.flex-item {
flex: 1 1 auto;
}
Alignment
- justify-content aligns items on the main axis
- align-items aligns items on the cross axis
.box {
display: flex;
align-items: center;
justify-content: center;
}
/* Basic keywords */
align-items: normal;
align-items: stretch;
/* Positional alignment */
/* align-items does not take left and right values */
align-items: center; /* Pack items around the center */
align-items: start; /* Pack items from the start */
align-items: end; /* Pack items from the end */
align-items: flex-start; /* Pack flex items from the start */
align-items: flex-end; /* Pack flex items from the end */
/* Positional alignment */
justify-content: center; /* Pack items around the center */
justify-content: start; /* Pack items from the start */
justify-content: end; /* Pack items from the end */
justify-content: flex-start; /* Pack flex items from the start */
justify-content: flex-end; /* Pack flex items from the end */
justify-content: left; /* Pack items from the left */
justify-content: right; /* Pack items from the right */
/* Baseline alignment */
/* justify-content does not take baseline values */
/* Normal alignment */
justify-content: normal;
/* Distributed alignment */
justify-content: space-between; /* Distribute items evenly
The first item is flush with the start,
the last is flush with the end */
justify-content: space-around; /* Distribute items evenly
Items have a half-size space
on either end */
justify-content: space-evenly; /* Distribute items evenly
Items have equal space around them */
justify-content: stretch; /* Distribute items evenly
Stretch 'auto'-sized items to fit
the container */
Grid
https://www.mozilla.org/en-US/developer/css-grid/
-
Grids are broken down into evenly spaced columns and rows that are used as a guide for laying out page components.
-
Gutters are often included to add consistent spacing between columns.
-
Page components are arranged within the columns.
-
grid container - the parent element.
-
grid items — the child elements within the grid container
<div class="grid-container">
<div class="grid-item">
<div>Not a grid item</div>
</div>
<div class="grid-item"></div>
<div class="grid-item"></div>
</div>
.grid-container {
display: grid;
/* OR */
display: inline-grid;
}
-
When using the value of grid, unless specified, the items will span the width of its container.
-
When using the value of grid, the container will be displayed as block-level element and stacked on top of other block element.
-
When using the value of inline-grid, the items will span the width of its content.
-
When using the value of inline-grid, the whole container will display inline next to other inline containers.
-
Grid lines are the horizontal and vertical lines that divide the grid into columns and rows.
- Grid lines are also used to determine the position of the grid items and are referred to by a numerical index, or by custom name.
- When using a numerical index, both the vertical and horizontal grid lines start at one.
- Grid lines can also be referred to by a negative numerical index which allows you to reference the opposite end of the grid.
-
A grid cell refers to a single unit, defined by where the grid row and column intersect.
- The space between two adjacent grid lines is the grid track, which is basically the columns and rows.
-
Grid tracks can optionally be separated by a gutter.
Explicit Grid
- To define a specific number of grid lines and tracks, use
grid-template-columns
andgrid-template-rows
. This will create an explicit grid.
.grid-container {
display: grid;
grid-template-columns: 100px 100px 100px; /* 3 columns */
grid-template-rows: 100px 100px; /* 2 rows */
}
The Fraction Unit: Fr
grid-template-columns: 1fr 1fr 1fr;
grid-template-columns: 1fr 2fr 1fr;
grid-template-columns: mixmax(200px, 1fr) 2fr 1fr; /* Set minimum allowed size */
repeat()
- We can also define multiple tracks using another value, the repeat function.
- Instead of writing 1 fr three times, include the number of tracks, three in this example, followed by a comma and the size of the track such as 1 fr within the parentheses.
repeat([number of tracks], [size of tracks])
grid-template-columns: 1fr 1fr 1fr;
/* same as */
grid-template-columns: repeat(3, 1fr);
grid-template-columns: 50px repeat(2, 1fr);
Adding Gutters with gap
gap: 10px; /* rows & columns */
gap: 10px 20px; /* rows | columns */
/* valid */
gap: 20px;
gap: 16%;
gap: calc(19% + 20px)
/* not valid */
gap: 1fr
/* shorthand */
gap: 110px 20px;
/* longhand */
row-gap: 10px;
column-gap: 20px;
- The original grid layout specification included the grid-gap, grid-row-gap and grid-column-gap properties.
- It has been updated to gap to be used for both grid and Flexbox.
/* shorthand */
gap = grid-gap
/* longhand */
row-gap = grid-row-gap
column-gap = grid-column-gap
Implicit Grid
- When using a grid layout, grid template rows and grid template columns are used to define a fixed number of tracks to form an explicit grid. But sometimes you may have more grid items than the number of explicit tracks.
- Once an explicit grid is filled or if no explicit grid has been defined, the grid container will generate implicit grid tracks.
- If you don’t know how many items your grid needs to display you can create the whole grid using only an implicit grid, and if you know the minimum amount of grid items you could use an explicit and implicit grid together.
grid-auto-columns grid-auto-rows .grid-container {
display: grid;
grid-auto-columns: repeat(3, 1fr); /* 3 columns */
grid-auto-rows: 100px 100px; /* 2 rows */
}
Defining the grid Structure
.grid-container {
display: grid;
grid-template-columns: 100px repeat(2, 1fr);
grid-template-rows: 100px;
grid-auto-rows: 200px;
grid-auto-columns: 50px;
}
Positioning grid Items
grid-column = grid-column-start grid-column-end grid-row = grid-row-start grid-row-end .grid-item-1 {
grid-column-start: 2;
grid-column-end: 4;
/* shorthand */
grid-column: 2 / 4;
grid-column: 1 / -1; /* span from start */
grid-row-start: 1;
grid-row-end: 3;
/*shorthand */
grid-row: 1 / 3;
grid-row: 1 / span 3;
}
Grid Lines and grid-column
.grid-item-1 {
grid-column-start: 2;
grid-column-end: 4;
/* shorthand */
grid-column: 2 / 4;
}
.grid-item-1 {
grid-column-start: 2;
grid-column-end: 4;
grid-row-start: 1;
grid-row-end: 3;
/* shorthand */
grid-column: 2 / 4;
grid-row: 1 / 3;
}
Alignment
- justify-items defines the default justify-self for all items of the box, giving them all a default way of justifying each box along the appropriate axis.
- align-items aligns items on the Block Axis within their grid area.
- place-items is shorthand property allows you to align items along both the block and inline directions at once (align-items and justify-items properties).
.grid {
display: grid;
grid-template-columns: repeat(auto-fill, 50px);
align-items: center;
justify-content: center;
justify-items: stretch;
}
- justify-content aligns items on the inline axis of a grid container.
- align-content sets the distribution of space between and around content items along grid’s block axis.
- place-content is shorthand property allows you to align content along both the block and inline directions at once (align-items and justify-items properties).
Naming the grid columns/rows
body {
grid-template-columns: [left] 1fr [middle] 1fr [right] 1fr;
}
header {
grid-column: left / right;
}
grid-template-areas
The grid-template-areas CSS property specifies named grid areas, establishing the cells in the grid and assigning them names.
<section id="page">
<header>Header</header>
<nav>Navigation</nav>
<main>Main area</main>
<footer>Footer</footer>
</section>
#page {
display: grid;
width: 100%;
height: 250px;
grid-template-areas:
"head head"
"nav main"
"nav foot";
grid-template-rows: 50px 1fr 30px;
grid-template-columns: 150px 1fr;
}
#page > header {
grid-area: head;
background-color: #8ca0ff;
}
#page > nav {
grid-area: nav;
background-color: #ffa08c;
}
#page > main {
grid-area: main;
background-color: #ffff64;
}
#page > footer {
grid-area: foot;
background-color: #8cffa0;
}
CSS Responsive Web Design
https://alistapart.com/article/responsive-web-design/
- Fluid layout
- Flexible images
- Media queries
Fluid Layouts
- Instead of fixed-width pixels, we’ll update our project to percentage-based values and use min and max width properties to create a flexible foundation.
- When the viewport is wider than the max width, the content wrap maxes out at the defined value, 800 pixels in this example.
- When the viewport is smaller than the max width value, it will apply the width value.
.content-wrap {
max-width: 800px;
width: 85%;
margin: auto;
padding: 60px 0;
}
Flexible Images
There’re two ways to add images to a webpage.
- Images that are part of the content should be added with HTML
<img src="1images/project-courses.jpg" alt="course thumbnail" />
- Images that are presentational should be added with CSS
section {
background-image: url(path/to/1image);
}
-
We’ve used the Shorthand Syntax background
to set the color, but the Longhand Properties actually background-color
.
-
background-image
is used to with a URL function value with the path to the image added within the parentheses.
-
If the image file is smaller than the area you applied it to, it will automatically repeat the image on the x and y-axis to fit the entire area. To keep the image from repeating, use the background-eepeat
Property set to no-repeat
.
-
By default, the background image is aligned to the top left of the container. background-position
can be used to define the position of the image relative to its container.
- One value represents the positioning from the left of the container.
- Any length value can be used.
- The top value defaults to auto, which will aligned the image vertically.
- Use a second value to specify the position from the top.
background-position: 100px 10%;
- There are also five keywords: top, bottom, left, right, and center.
-
To get the image to cover the entire area without repeating, background-size
can be used to set the width and the height.
- The first value is applied to the width.
- Use a second value to change the height of the image.
background-size: 100% 100%;
-
A 100% will make it stretch all the way across and is relative to its container.
-
Another option is to use the keyword cover
. To maintain the aspect ratio, the part of the image that doesn’t fit into the container will be clipped.
background-size: cover;
- This is not a perfect solution, especially if an important part of the image is not shown. We can use the Background Position Property to move a specific part of the image within the viewable part of the container.
background-position: top right;
.background {
max-width: 600px;
padding: 100px;
/* background-color: lightblue; */
/* background-image: url(https://unsplash.it/550/150?image=703); */
/* background-repeat: no-repeat; */
/* background-position: 100px; */
/* background-size: 100%; */
}
background
Shorthand
background-size
may only be included after position, separated with the ’/’ character
selector {
/* image, repeat style, position, background size */
background: url(../image.jpg) no-repeat center/cover;
- background-size may be declared independently
selector {
background: blue url(image.jpg) no-repeat;
background-size: cover;
}
selector {
background-color: red; /* value will be overwritten */
}
.more-specific-selector {
background: url(images/pic.png) no-repeat;
}
More Info: https://developer.mozilla.org/en-US/docs/Web/CSS/background
Media Queries
-
Media queries make it possible to modify the CSS depending on specific conditions, defined with a media type and a media feature.
-
Media queries are added to the CSS file using the @media rule.
-
In this example, this query translates to: when the browser view port is smaller than 1000 pixels, apply this block of CSS. If this condition is not met, the CSS is ignored.
@media screen and (max-width: 1000px) {
hl {
font-size: 16px;
}
}
Media Types
Declaring the media type is optional, so if you don’t include it, it will default to all.
- all: matches to all devices
- print: matches to printers and print-related displays
- speech: matches to screen reading devices that “read out” a page
- screen: matches all devices that aren’t categorized as print or speech
@media all {
...;
}
@media print {
...;
}
@media speech {
...;
}
@media screen {
...;
}
Media Features
- Media features are used to test a specific feature of the device
@media (width: 480px) {
...;
}
@media (orientation: landscape) {
...;
}
- Use keyword “and” to combine media features with media types
@media (orientation: landscape) {
...;
}
@media screen and (orientation: landscape) {
...;
}
@media screen and (min-width: 30em) and (orientation: landscape) {
...;
}
@media (orientation: landscape) and (min-width: 320px) {
...;
}
- There are more logical operators, such as “not” or “only.”
- There are also quite a few media features: width, height, aspect ratio, and more.
More info: https://developer.mozilla.org/en-US/docs/Web/CSS/Media_Queries/Using_media_queries
Where do we include the media query?
- It’s convention to either add all the media queries at the bottom of the CSS file.
hl {
font-size: 16px;
}
h2 {
font-size: 1l4px;
}
@media (max-width: 1000px) {
hl {
font-size: 12px;
}
}
- Or add the media query beneath the related CSS style.
- This way of adding media queries is a little more commonly used when writing CSS with preprocessors such as Sass or Less.
hl {
font-size: 16px;
}
@media max-width: {
hl {
font-size: 12px;
}
}
h2 {
font-size: 1l4px;
}
-
You can also write all your media queries in a separate style sheet, and then load them into the main CSS file.
- Use @import to load the stylesheet into the main CSS file.
@import url("mobile.css") screen and (max-width: 480px);
- Use
<link>
to load the stylesheet into the HTML file.
<link rel="stylesheet" media="screen and (max-width: 480px)" href="mobile.css" />
Breakpoints
- 320px: Mobile portrait
- 480px: Mobile landscape
- 600px: Small tablet
- 768px: Tablet portrait
- 940-1024px: Tablet landscape
- 1280px and greater: Desktop, laptop
Width Media Feature
- When using the Width Media Feature, the media query test applies to the width of the browser viewport.
- Width is classified as a range feature, meaning that we can also use a min or max prefix to specify a minimum or maximum value.
- Using the min or max value works better than just width because it specifies a range such as larger than or smaller than.
- If we use width alone, then it only applies if the viewport is exactly equal to the defined value.
/* Exact width */
@media (width: 360px) {
...;
}
/* Minimum width - 360px or larger */
@media (min-width: 360px) {
...;
}
/* Maximum width - 360px or smaller */
@media (max-width: 360px) {
...;
}
min-
and max-width
Breakpoints
- Sometimes when you’re using both min and max-width values, you may end up targeting the same resolution.
- A simple solution for this would be just to make one of the values one pixel smaller or bigger.
/* 800px and larger */
@media (min-width: 800px) {
...;
}
/* 799px and smaller */
@media (max-width: 799px) {
...;
}
/* OR */
/* 801px and larger */
@media (min-width: 801px) {
...;
}
/* 800px and smaller */
@media (max-width: 800px) {
...;
}
Designing for different screens
There are two schools of thought when designing for different screens.
- One is Desktop First, meaning you design and write your CSS for bigger screens first.
- Media queries are then used to make changes for smaller screens.
- This approach usually uses max-width media queries.
/* Optimized for larger screens */
body {
...;
}
/* Changes made for smaller screens x*/
@media (max-width: 800px) {
body {
...;
}
}
@media (max-width: 400px) {
body {
...;
}
}
- A Mobile First approach is the opposite.
- The designs in CSS cater to smaller sizes first.
- Media queries are then used to make changes for bigger screens.
- This approach usually uses min-width media queries.
/* Optimized for smaller screens */
body {
...;
}
/* Changes made for larger screens */
@media (min-width: 800px) {
body {
...;
}
}
@media (min-width: 1200px) {
body {
...;
}
}
You can also combine both min and max to create a range.
/* This applies a range of 400px to 800px */
@media (min-width: 400px) and (max-width: 800px) {
body {
...;
}
}
viewport meta tag
- There’s one more thing you need to make sure is included before we finally get to adding media queries. The viewport meta tag.
- This is added to the head section of the HTML document, and is used to control the viewport’s size and scale and optimize the document for mobile viewing.
- The values in the content attribute set the page to render the same width as the device.
- Also, setting the initial scale to one, just means that the page won’t appear zoomed in or out on page load.
- More: https://developer.mozilla.org/en-US/docs/Mozilla/Mobile/Viewport_meta_tag
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
وصلة للملاحظة الرئيسة
CSS Responsive Web Design
https://alistapart.com/article/responsive-web-design/
- Fluid layout
- Flexible images
- Media queries
Fluid Layouts
- Instead of fixed-width pixels, we’ll update our project to percentage-based values and use min and max width properties to create a flexible foundation.
- When the viewport is wider than the max width, the content wrap maxes out at the defined value, 800 pixels in this example.
- When the viewport is smaller than the max width value, it will apply the width value.
.content-wrap {
max-width: 800px;
width: 85%;
margin: auto;
padding: 60px 0;
}
Flexible Images
There’re two ways to add images to a webpage.
- Images that are part of the content should be added with HTML
<img src="1images/project-courses.jpg" alt="course thumbnail" />
- Images that are presentational should be added with CSS
section {
background-image: url(path/to/1image);
}
-
We’ve used the Shorthand Syntax
background
to set the color, but the Longhand Properties actuallybackground-color
. -
background-image
is used to with a URL function value with the path to the image added within the parentheses. -
If the image file is smaller than the area you applied it to, it will automatically repeat the image on the x and y-axis to fit the entire area. To keep the image from repeating, use the
background-eepeat
Property set tono-repeat
. -
By default, the background image is aligned to the top left of the container.
background-position
can be used to define the position of the image relative to its container.- One value represents the positioning from the left of the container.
- Any length value can be used.
- The top value defaults to auto, which will aligned the image vertically.
- Use a second value to specify the position from the top.
background-position: 100px 10%;
- There are also five keywords: top, bottom, left, right, and center.
-
To get the image to cover the entire area without repeating,
background-size
can be used to set the width and the height.- The first value is applied to the width.
- Use a second value to change the height of the image.
background-size: 100% 100%;
-
A 100% will make it stretch all the way across and is relative to its container.
-
Another option is to use the keyword
cover
. To maintain the aspect ratio, the part of the image that doesn’t fit into the container will be clipped.background-size: cover;
- This is not a perfect solution, especially if an important part of the image is not shown. We can use the Background Position Property to move a specific part of the image within the viewable part of the container.
background-position: top right;
.background {
max-width: 600px;
padding: 100px;
/* background-color: lightblue; */
/* background-image: url(https://unsplash.it/550/150?image=703); */
/* background-repeat: no-repeat; */
/* background-position: 100px; */
/* background-size: 100%; */
}
background
Shorthand
background-size
may only be included after position, separated with the ’/’ character
selector {
/* image, repeat style, position, background size */
background: url(../image.jpg) no-repeat center/cover;
- background-size may be declared independently
selector {
background: blue url(image.jpg) no-repeat;
background-size: cover;
}
selector {
background-color: red; /* value will be overwritten */
}
.more-specific-selector {
background: url(images/pic.png) no-repeat;
}
More Info: https://developer.mozilla.org/en-US/docs/Web/CSS/background
Media Queries
-
Media queries make it possible to modify the CSS depending on specific conditions, defined with a media type and a media feature.
-
Media queries are added to the CSS file using the @media rule.
-
In this example, this query translates to: when the browser view port is smaller than 1000 pixels, apply this block of CSS. If this condition is not met, the CSS is ignored.
@media screen and (max-width: 1000px) {
hl {
font-size: 16px;
}
}
Media Types
Declaring the media type is optional, so if you don’t include it, it will default to all.
- all: matches to all devices
- print: matches to printers and print-related displays
- speech: matches to screen reading devices that “read out” a page
- screen: matches all devices that aren’t categorized as print or speech
@media all {
...;
}
@media print {
...;
}
@media speech {
...;
}
@media screen {
...;
}
Media Features
- Media features are used to test a specific feature of the device
@media (width: 480px) {
...;
}
@media (orientation: landscape) {
...;
}
- Use keyword “and” to combine media features with media types
@media (orientation: landscape) {
...;
}
@media screen and (orientation: landscape) {
...;
}
@media screen and (min-width: 30em) and (orientation: landscape) {
...;
}
@media (orientation: landscape) and (min-width: 320px) {
...;
}
- There are more logical operators, such as “not” or “only.”
- There are also quite a few media features: width, height, aspect ratio, and more.
More info: https://developer.mozilla.org/en-US/docs/Web/CSS/Media_Queries/Using_media_queries
Where do we include the media query?
- It’s convention to either add all the media queries at the bottom of the CSS file.
hl {
font-size: 16px;
}
h2 {
font-size: 1l4px;
}
@media (max-width: 1000px) {
hl {
font-size: 12px;
}
}
- Or add the media query beneath the related CSS style.
- This way of adding media queries is a little more commonly used when writing CSS with preprocessors such as Sass or Less.
hl {
font-size: 16px;
}
@media max-width: {
hl {
font-size: 12px;
}
}
h2 {
font-size: 1l4px;
}
-
You can also write all your media queries in a separate style sheet, and then load them into the main CSS file.
- Use @import to load the stylesheet into the main CSS file.
@import url("mobile.css") screen and (max-width: 480px);
- Use
<link>
to load the stylesheet into the HTML file.
<link rel="stylesheet" media="screen and (max-width: 480px)" href="mobile.css" />
Breakpoints
- 320px: Mobile portrait
- 480px: Mobile landscape
- 600px: Small tablet
- 768px: Tablet portrait
- 940-1024px: Tablet landscape
- 1280px and greater: Desktop, laptop
Width Media Feature
- When using the Width Media Feature, the media query test applies to the width of the browser viewport.
- Width is classified as a range feature, meaning that we can also use a min or max prefix to specify a minimum or maximum value.
- Using the min or max value works better than just width because it specifies a range such as larger than or smaller than.
- If we use width alone, then it only applies if the viewport is exactly equal to the defined value.
/* Exact width */
@media (width: 360px) {
...;
}
/* Minimum width - 360px or larger */
@media (min-width: 360px) {
...;
}
/* Maximum width - 360px or smaller */
@media (max-width: 360px) {
...;
}
min-
and max-width
Breakpoints
- Sometimes when you’re using both min and max-width values, you may end up targeting the same resolution.
- A simple solution for this would be just to make one of the values one pixel smaller or bigger.
/* 800px and larger */
@media (min-width: 800px) {
...;
}
/* 799px and smaller */
@media (max-width: 799px) {
...;
}
/* OR */
/* 801px and larger */
@media (min-width: 801px) {
...;
}
/* 800px and smaller */
@media (max-width: 800px) {
...;
}
Designing for different screens
There are two schools of thought when designing for different screens.
- One is Desktop First, meaning you design and write your CSS for bigger screens first.
- Media queries are then used to make changes for smaller screens.
- This approach usually uses max-width media queries.
/* Optimized for larger screens */
body {
...;
}
/* Changes made for smaller screens x*/
@media (max-width: 800px) {
body {
...;
}
}
@media (max-width: 400px) {
body {
...;
}
}
- A Mobile First approach is the opposite.
- The designs in CSS cater to smaller sizes first.
- Media queries are then used to make changes for bigger screens.
- This approach usually uses min-width media queries.
/* Optimized for smaller screens */
body {
...;
}
/* Changes made for larger screens */
@media (min-width: 800px) {
body {
...;
}
}
@media (min-width: 1200px) {
body {
...;
}
}
You can also combine both min and max to create a range.
/* This applies a range of 400px to 800px */
@media (min-width: 400px) and (max-width: 800px) {
body {
...;
}
}
viewport meta tag
- There’s one more thing you need to make sure is included before we finally get to adding media queries. The viewport meta tag.
- This is added to the head section of the HTML document, and is used to control the viewport’s size and scale and optimize the document for mobile viewing.
- The values in the content attribute set the page to render the same width as the device.
- Also, setting the initial scale to one, just means that the page won’t appear zoomed in or out on page load.
- More: https://developer.mozilla.org/en-US/docs/Mozilla/Mobile/Viewport_meta_tag
<meta name="viewport" content="width=device-width, initial-scale=1.0" />